Building Micronaut Microservices Using MicrostarterCLI: A Comprehensive Guide
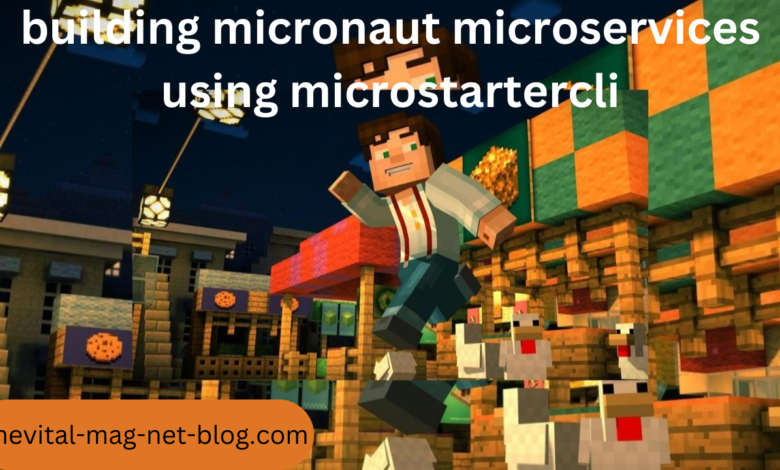
Micronaut is a powerful, modern framework for building microservices. Its design focuses on providing high performance, fast startup times, and a lightweight footprint, which makes it ideal for building scalable, cloud-native applications. One of the most efficient ways to get started with Micronaut microservices is by using the microstartercli
, a command-line tool that simplifies the process of creating and managing Micronaut-based applications.
This article will guide you through the process of building Micronaut microservices using microstartercli
, explaining the key concepts, steps, and best practices for setting up your environment and creating production-ready microservices.
What is Micronaut?
Micronaut is a modern JVM-based framework designed specifically for building microservices and serverless applications. Unlike traditional frameworks, Micronaut doesn’t rely on reflection, which makes it highly efficient and ideal for microservices, especially in resource-constrained environments.
Some of the key features of Micronaut include:
- Dependency Injection: A core feature of Micronaut is its built-in, compile-time dependency injection, which eliminates the overhead of runtime reflection.
- Aspect-Oriented Programming (AOP): Micronaut supports AOP, which allows developers to modularize cross-cutting concerns like logging, security, and monitoring.
- Cloud-Native: The framework is designed for cloud environments with built-in support for Kubernetes, service discovery, and centralized configuration.
- Fast Startup Time: Micronaut is optimized for low latency and fast startup times, making it suitable for serverless environments.
- Microservices: Micronaut provides tools and features to build and deploy microservices with minimal setup.
What is MicrostarterCLI?
microstartercli
is a command-line tool created by the Micronaut team to simplify the process of bootstrapping new Micronaut projects. It allows you to quickly generate a base application that’s preconfigured with essential libraries and configurations to start building your microservices.
The tool helps streamline the setup process by asking for minimal user input, making it easy to configure your application, integrate with various services, and get started with Micronaut in minutes. Using microstartercli
, developers can generate a ready-to-use Micronaut application with the necessary dependencies for common tasks such as database integration, security, and more.
Prerequisites for Using MicrostarterCLI
Before you can start building Micronaut microservices using microstartercli
, there are a few prerequisites you need to have in place:
- Java JDK: Micronaut is based on Java, so you’ll need a version of the Java Development Kit (JDK) installed on your machine. Micronaut supports JDK 8 and above.
- Micronaut CLI: You’ll need to install the Micronaut CLI, which includes the
microstartercli
tool. - Maven or Gradle: Depending on your project’s build system, you’ll need either Maven or Gradle to handle project dependencies and build configurations.
- IDE: While not strictly required, using an Integrated Development Environment (IDE) like IntelliJ IDEA or Visual Studio Code can make development smoother.
Installing Micronaut and MicrostarterCLI
To begin, you need to install Micronaut and the microstartercli
. Here’s how you can do it:
- Install Micronaut CLI: On macOS or Linux, you can install Micronaut using SDKMAN by running the following commands:
sdk install micronaut
On Windows, you can download the latest version of Micronaut from the official Micronaut website. - Install microstartercli: Once Micronaut CLI is installed,
microstartercli
should already be included. You can confirm this by running:micronaut --help
Building Micronaut Microservices Using MicrostarterCLI
Now that you’ve installed the necessary tools, let’s go through the steps of building a Micronaut-based microservice using microstartercli
.
Step 1: Creating a New Micronaut Project
To start a new Micronaut project, you can use the create-app
command from microstartercli
. This command will scaffold a new application with basic configurations and dependencies that you can build on. Here’s how to use the create-app
command:
micronaut create-app com.example.microservice --features http-client,hibernate-jpa
In this example:
com.example.microservice
is the group ID and artifact ID of your project.--features
specifies additional features or modules that you want to include in your application, such as an HTTP client or Hibernate for JPA.
You can specify any additional features you need for your project, such as security, messaging, or logging, based on your requirements.
Step 2: Understanding the Project Structure
Once the create-app
command completes, a new project folder will be generated. Here’s an overview of the important directories and files in the Micronaut project:
- src/main/java: This directory contains the Java classes for your microservice.
- src/main/resources: This directory contains configuration files such as
application.yml
, which controls application-level settings like server port, logging, etc. - src/test/java: This directory contains test classes for your application.
Micronaut projects follow a clean separation of concerns, where each module or service can be independently tested and maintained.
Step 3: Developing the Microservice
With the basic project in place, you can now start developing the microservice. A simple example would be to create a REST controller to handle HTTP requests.
For instance, let’s create a controller that handles GET
requests:
package com.example.microservice;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
@Controller("/hello")
public class HelloController {
@Get
public String hello() {
return "Hello, Micronaut!";
}
}
This controller will respond with “Hello, Micronaut!” when the /hello
endpoint is accessed. Micronaut’s HTTP server will automatically handle routing, serialization, and error management.
Step 4: Running the Microservice
Once you have your microservice logic in place, you can run the application using the Micronaut CLI:
./gradlew run
Or if you are using Maven:
./mvnw mn:run
This will start your Micronaut application on the default port (usually 8080). You can test the /hello
endpoint by visiting http://localhost:8080/hello
in your browser or using a tool like Postman or curl.
Step 5: Adding Persistence and Database Integration
Most microservices need some form of data persistence. Micronaut integrates well with several database technologies, such as MySQL, PostgreSQL, and MongoDB. You can use the hibernate-jpa
feature to enable JPA support in your application.
For example, you can create an entity class like this:
package com.example.microservice;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Person {
@Id
private Long id;
private String name;
private String email;
// getters and setters
}
You can then inject a repository to manage the entity:
package com.example.microservice;
import io.micronaut.data.annotation.Repository;
import io.micronaut.data.repository.CrudRepository;
@Repository
public interface PersonRepository extends CrudRepository<Person, Long> {
}
Now, you can use PersonRepository
to perform CRUD operations within your microservice.
Step 6: Deploying the Microservice
Once your microservice is ready, it’s time to deploy it. Micronaut supports various deployment options such as Docker, Kubernetes, and serverless platforms. You can package your application as a Docker image:
- Create a Dockerfile in the project root:
FROM openjdk:17-jdk-slim
COPY build/libs/*.jar app.jar
ENTRYPOINT ["java", "-jar", "/app.jar"]
- Build the Docker image:
docker build -t my-micronaut-microservice .
- Run the Docker container:
docker run -p 8080:8080 my-micronaut-microservice
Your microservice is now running inside a Docker container and is ready for deployment to any cloud or on-premise infrastructure.
Conclusion
Building Micronaut microservices using microstartercli
is a fast, efficient, and flexible way to get started with modern microservices architecture. The combination of Micronaut’s features and microstartercli
‘s ease of use allows developers to focus on building business logic instead of boilerplate setup. From creating a new project to deploying it in production, microstartercli
simplifies the workflow, making it an excellent tool for developing scalable and high-performance microservices.
Whether you’re working on a small service or a large microservices-based application, Micronaut, combined with microstartercli
, provides the right foundation for building cloud-native applications.